Training an Image-to-Text Translation Model with Python
Learn how to train an Image-to-Text Translation model using Python. This step-by-step guide covers everything from installing necessary libraries (OpenCV, Pytesseract, GoogleTrans) to pre-processing images, extracting text, and translating it between languages. Ideal for developers and tech enthusiasts looking to automate image translations efficiently.
Most of us already know that Python is an object-oriented high-level programming language. It is widely used for training models, software, tools, etc. so that they can quickly and efficiently perform automated tasks. Today, in this blog we are going to train a specific type of model known as an “Image-to-text Translator” with Python. Once the image translator is trained, it will have the ability to translate text pictures from one language to another within seconds. So, without discussing any additional info, let’s head towards the steps.
How to Train an Image-to-Text Translation Model Through Python
Below are the steps that you need to follow to efficiently train an image translation model with Python. Download & Install the Required Libraries First: To train an image translator using Python, you first have to install the required libraries on your PC or laptop. OpenCV: It is an open-source Python library for machine learning, image processing, and computer vision. Pytesseract: It is an Optical Character Recognition library that helps Python algorithms quickly and efficiently extract data from images. GoogleTrans: It is also a Python library that uses Google Translate Ajax APIs. This library will play a key role in the training process. You should download the latest version of Python; it will contain both OpenCV and Pytesseract libraries in it. When it comes to GoogleTrans, you can get this library here. Import the libraries Once you are done with downloading and installing libraries, you then have to import libraries to make them work during the training process. Below is the Python code that you need to write in your code editor.
import cv2
import py-tesseract
import googletrans
from googletrans.exceptions import RequestError
Pre-process the input image (Optional):
After importing all the required libraries, you should upload the required image and imply pre-processing on it. This is an option step, but it would be good if you do.
This is because, in image processing, all the distortion and noises will be removed by the installed Python libraries, making the input picture completely grayscale.
The grayscale conversion will make it easier for Pytesseract and GoogleTrans to efficiently extract and translate the given text.
Below is the code through which you can kick off the image pre-processing.
def preprocess_image(img):
Preprocesses an image (optional) to enhance text clarity.
Args:
img: The image as a NumPy array.
Returns:
The preprocessed image as a NumPy array.
# Example: Convert to grayscale
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
return gray
Start The Image Reading Process:
The next step is to start the image reading process for translation. This process will be performed by the CV2 library, previously known as OpenCV2.
During this step, the CV2 library will make sure whether the input picture is perfectly readable or not. If it is readable, the Python algorithms will move it toward the extraction process.
On the other hand, if the image is not properly readable or contains any kind of error, then Image to text translation model will display “Error: Could not read image at the given path.” The Python code you will need to perform this step is below.
def read_image(image_path):
Reads an image from a specified path.
Args:
image_path: Path to the image file.
Returns:
The loaded image is a NumPy array.
img = cv2.imread(image_path)
if img is None:
print(f"Error: Could not read image at {image_path}")
return img
Extract Text
The name of this step says it at. Once the model has efficiently fetched the required picture from the given text, you then have to train it for text extraction. For this, Pytesseract OCR will play a key role. The code you will need is below.
def extract_text(img):
Extracts text from an image using Tesseract OCR.
Args:
img: The image as a NumPy array (grayscale recommended).
Returns:
Extracted text as a string.
# Improve accuracy by configuring Tesseract with configs (adjust as needed)
config = '--psm 6' # Treat image as a single block of text
text = pytesseract.image_to_string(img, config=config)
return text
Translate The Text:
Finally, you then have to integrate the Google Translate library into the image-to-text translation model. So that, it can get the ability to quickly and efficiently translate the extracted text from one language to another.
def translate_text(text, target_lang='en'):
Translates text to a target language using Google Translate API.
Args:
text: Text to be translated.
target_lang: Target language code (default: English).
Returns:
Translated text as a string. Handles potential translation errors.
translator = googletrans.Translator()
try:
translated = translator.translate(text, dest=target_lang)
return translated.text
except RequestError as e:
print(f"Translation error: {e}")
return None
These are steps that you need to follow to train an image translator model with Python. However, keep in mind that, if your code contains a single mistake, you may run into an error, if not, then the model you trained may not be able to work properly. So, BE CAREFUL WHILE WRITING PYTHON CODE! Final Words Python is a high-level programming language that is widely used to train applications, software, or tools. So that they can perform automated tasks. In this article, we have explained the step-by-step training procedure of one such model known as an image-to-text translator. We are quite hopeful that you will find this article valuable.
Experience the full potential of ChatGPT with Merlin
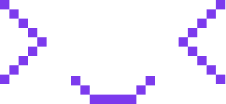
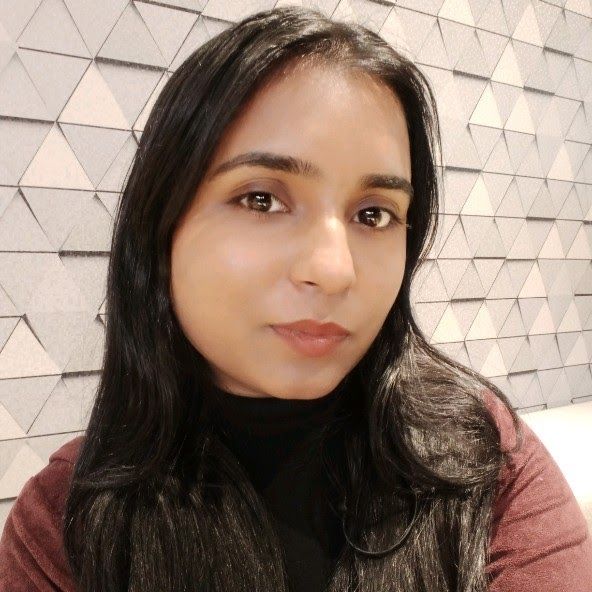
Kalpna Thakur
Our marketing powerhouse, crafts innovative solutions for every growth challenge - all while keeping the fun in our team!